Arduino sketch and data transmission
On EFFE’s blog, I found RFM01 RFM02 Arduino libraries supporting the above wiring scheme. The libraries support transmission of a short, 16 char text string from one Arduino to the other, which was very suitable for my temperature sensor project.
Since the 433 MHz is used by many devices, such as remote power sockets, weather stations etc., this frequency is full of “noise”. Therefore it was necessery to implement a simple software-based error correction protocol: In order to mark the begin of a data packet, the string “AAAA” is sent, followed by a string containing the temperature (e.g. “2095” for 20.95 degrees celsius). The string is stored as a variable of type “char”, so that one can calculate a simple “checksum” by adding the ASCII decimal values of it’s four digits. When the temperature data arrives on the receiver, it’s checksum is compared with the checksum sent by the transmitter. If both match, the temperature is displayed on the 2×16 LCD.
// RF02 transmitter sketch, DALLAS DS18S20 1-wire temperature sensor #include <rf02.h> #include <stdint.h> #include <DallasTemperature.h> #include <OneWire.h> #define ONE_WIRE_BUS 6 //D10 OneWire oneWire(ONE_WIRE_BUS); DallasTemperature sensors(&oneWire); void setup() { rf02_prepAll434(); pinMode(4, OUTPUT); pinMode(5, OUTPUT); ledBlink(4); ledBlink(5); } void ledBlink(int num){ digitalWrite(num, HIGH); delay(100); digitalWrite(num, LOW); delay(100); } void loop() { ledBlink(4); sensors.begin(); sensors.requestTemperatures(); int temp = sensors.getTempCByIndex(0)*100; char head[5]="AAAA"; char msg[8]; itoa(temp, msg,10); int sum; int i; for (i=0; i<=3; i++){ sum += msg[i]; } char chk[5]; itoa(sum, chk ,10); rf02_changeText( head, sizeof head); rf02_sendData(); delay(1000); rf02_changeText( msg, sizeof msg); rf02_sendData(); delay(1000); rf02_changeText( chk, sizeof chk); rf02_sendData(); ledBlink(5); delay(1000);
Over a distance of about 15 m, even through a closed balcony door, it takes only about 2 to 5 attempts for a successful transmission (about five to twenty seconds). Thus, it is reasonable to continue with these modules to build a larger project including more sensors or transmitter modules.
// RF01 receiver sketch, DALLAS DS18S20 1-wire temperature sensor #include <LiquidCrystal595.h> // 16x2 LCD backpack with 74HC595 shift register #include <rf01.h> LiquidCrystal595 lcd( 5, 6, 7); // pins for Atmega328/ Arduino int serialTesting = 0; int j; void setup() { lcd.begin(16,2); // init LCD display lcd.setCursor(2,0); // place the cursor at row1 col2 lcd.print("DS18S20"); // print a message lcd.setCursor(3,1); lcd.print("Thermometer"); delay(1000); lcd.clear(); rf01_prepAll(); delay(1000); } void loop() { rf01_receive(); // Listen at 433 MHz char* buf = (char*) rf01_data; // catch first data packet (begin) if ( buf = "AAAA" ) { // AAAA marks begin of data packet rf01_receive(); // catch next data packet (temperature) char* buf = (char*) rf01_data; int sum; // calculate a simple checksum1 int i; for (i=0; i<=3; i++){ sum += buf[i]; } lcd.setCursor(1,1); lcd.print("Sum: "); lcd.print(sum); lcd.print(" "); float tempC = atof(buf) / 100; rf01_receive(); // catch next data packet (checksum2 calculated on RF02) char* buf1 = (char*) rf01_data;; if (sum == atoi(buf1)) { // compare checksum1 and checksum2 and print to LCD if they match lcd.setCursor(1,0); lcd.print("Temp: "); lcd.print(tempC); lcd.print(" C "); j = 0; } if (sum != atoi(buf1)) { j +=1; lcd.setCursor(12,1); lcd.print(j); lcd.print(" "); } } delay(1000);
If you want to connect more sensors or want to use more RF02 transmitters, simply include a new “head” tag for each device in the sketch. This will allow to distinguish between different sensors or transmitter modules.
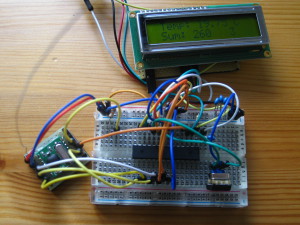