Reading the temperature signal of LM335Z from analog input
The LM335 has a breakdown voltage directly proportional to absolute temperature at +10 mV/Kelvin. It has to be connected to an analog pin of the Arduino as shown in the diagram below.
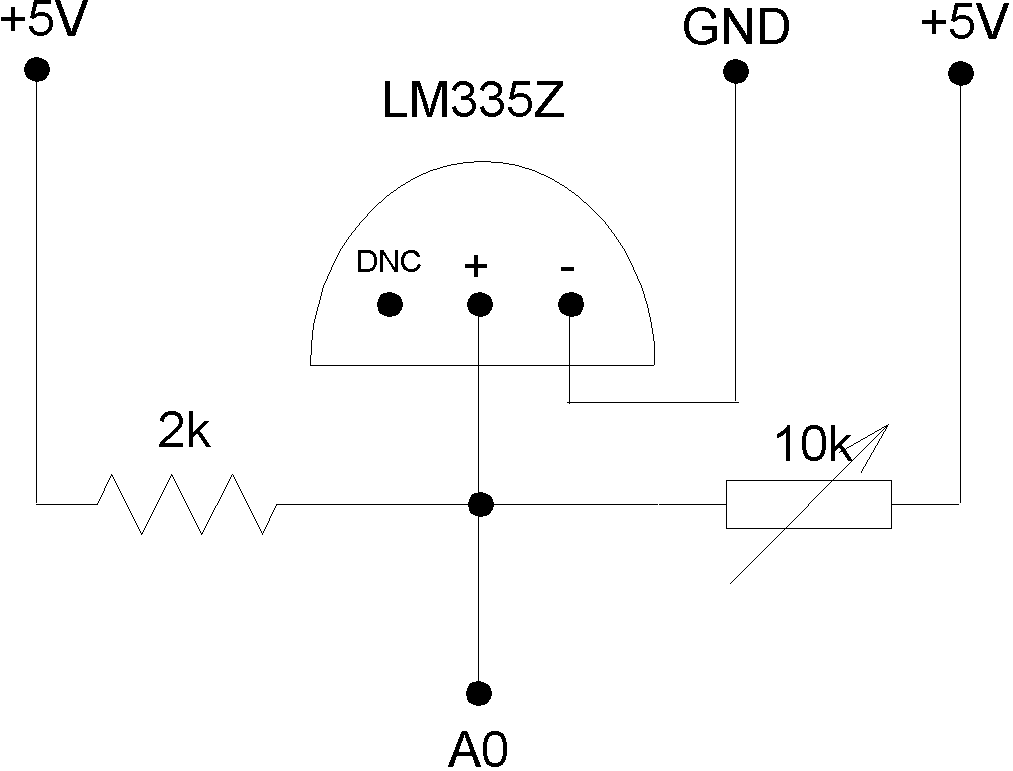
Arduino sketch
The sketch to read the temperature from LM335Z consists of four major parts. First, we define the pins that are used for serial transfer of the data to the shift register (DATA, LATCH and CLOCK). Then, we define an array which contains the bits patterns representing the digits 0 to 9 to be shown on the 7-segment display. The absolute temperature (tK) is read from the sensor as an analog signal and is converted to degrees Celsius (temp). The first and second digit of the current temperature is then converted to an integer value (d1, d2). Finally, the bit patterns representing each digit are transfered to the display with the last significant bit first (LSBFIRST).
const byte LATCH_PIN = 10; // connect to shift register RCK pin const byte DATA_PIN = 11; // connect to shift register SI pin const byte CLOCK_PIN = 13; // connect to shift register SCK pin const int TEMP_PIN = A0; // Arduino A0 // Bit array to represent digits on the 7-segment display // a b c d e f dp const byte digit[] = { 0B00000011, // 0 0B10011111, // 1 0B00100101, // 2 0B00001101, // 3 0B10011001, // 4 0B01001001, // 5 0B01000001, // 6 0B00011111, // 7 0B00000001, // 8 0B00001001, // 9 0B00000000, // all on }; void setup() { pinMode(LATCH_PIN, OUTPUT); pinMode(DATA_PIN, OUTPUT); pinMode(CLOCK_PIN, OUTPUT); pinMode(TEMP_PIN, INPUT); Serial.begin(9600); } // The temperature is read in degrees Kelvin, thus we convert // it into Celsius before output on the display void loop() { float tK = analogRead(0) * 5.0 / 1024 * 100; int temp = (int)tK - 273.15; //Serial.println(temp); int d1 = temp {c7f7cb1468c0d02af358b3ce02b96b7aadc0ce32ccb53258bc8958c0e25c05c4} 10; //Serial.println(d1); temp = temp/10; int d2 = temp {c7f7cb1468c0d02af358b3ce02b96b7aadc0ce32ccb53258bc8958c0e25c05c4} 10; //Serial.println(d2); displayTemp(d1, d2); delay(1000); // delay for updating the display (milliseconds), lower values // may result in flickering } void displayTemp(int a, int b){ byte bitsA = digit[a]; byte bitsB = digit[b]; digitalWrite(LATCH_PIN, LOW); shiftOut(DATA_PIN, CLOCK_PIN, LSBFIRST, bitsA); shiftOut(DATA_PIN, CLOCK_PIN, LSBFIRST, bitsB); digitalWrite(LATCH_PIN, HIGH); }